Almost all of us have had to start something new, be it a new job or a new school. When I find myself in this situation, I make it my mission to do two things:
- Get to know as many people as possible
- Find out what tech they are using
Fortunately, n8n has a great way of doing both at once. Using this coffee chat workflow, I can familiarize myself with their systems and get to meet a bunch of people at the same time.
This workflow automatically arranges coffee chats with people in Mattermost. It even schedules the appointment in their calendars! Virtual networking has never been so easy.
In this article, we are going to learn how to build this workflow and then change it to accommodate different chat systems that you may find plus accommodate some differences in the technology.
Prerequisites
This article assumes that you have the following in place already:
n8n
You will need a working copy of n8n. This can be either installed on your system or running on the new n8n cloud environment. This workflow was created in n8n version 0.101.0.
Google Calendar
We will be using Google Calendar to send out the meeting requests. It is important that you have access to this online app and that you have already set up the credentials in n8n as outlined here.
Chat Program
You will need to have one of the two following chat programs:
Please ensure that you have created accounts for one of these programs and set up the credentials for n8n to access them. If you need more information on setting up the credentials for these chat systems, please check out the appropriate set of documentation below:
Quick Start
If you are just here to get this workflow up and running and are not interested in figuring out what each of the nodes does, you can take a shortcut and download the already completed workflow located here:
Please select the one that matches the technology and accounts that you have. All you will be required to do is paste the workflow into your copy of n8n and then modify the corresponding nodes for the chat system and Google Calendar.
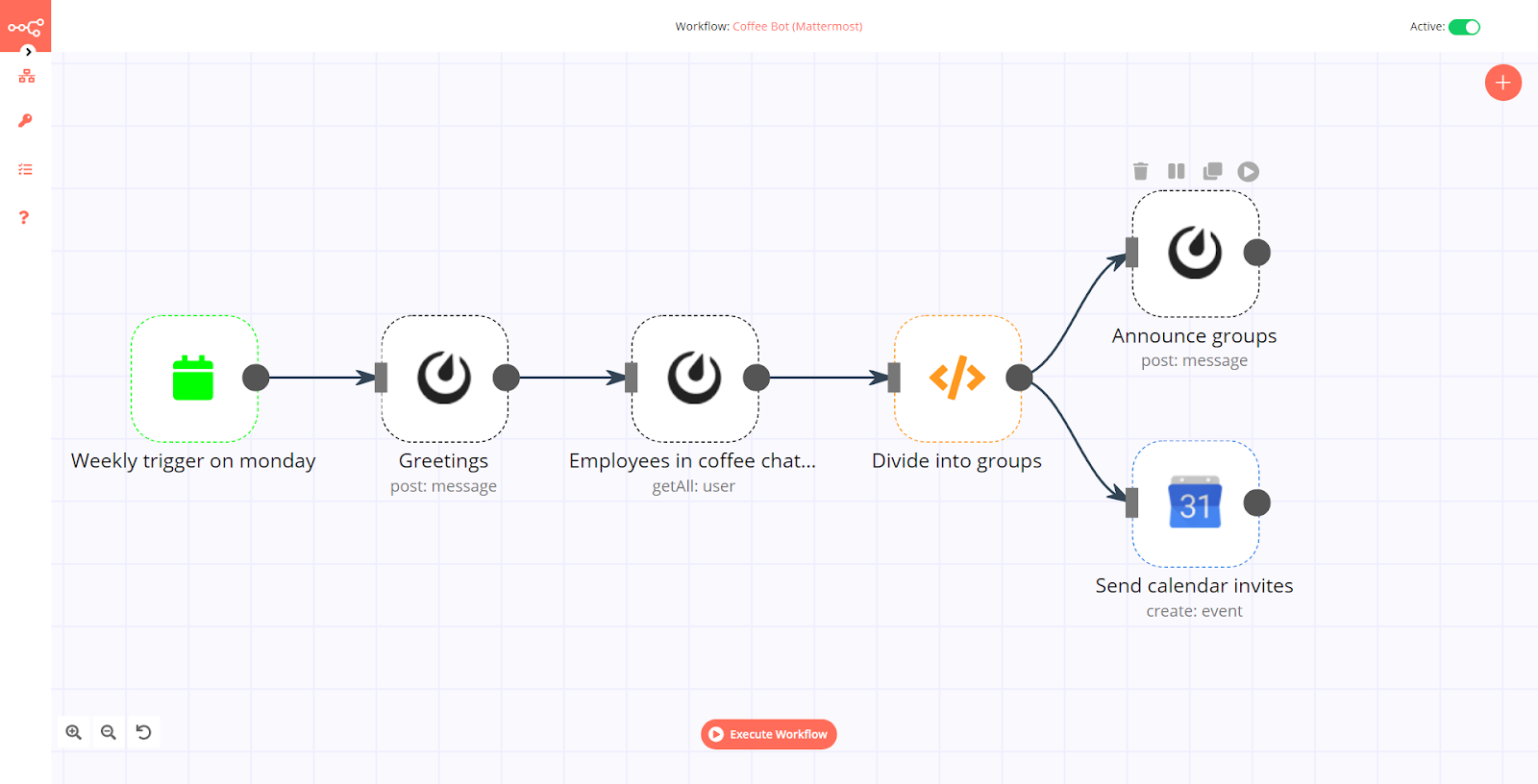
The Mattermost Workflow
There are four different nodes used in this workflow:
Cron Node
We would like this workflow to run once a week each Monday. To accomplish that, we use the cron node. To configure this to run at 10:00 each Monday morning, we will set the node parameters as follows:
- Mode: Every Week
- Hour: 10
- Minute: 0
- Weekday: Monday
Once you set each of these parameters, you can rename the cron node to Weekly Trigger on Monday and close the node.
First Mattermost Node (Greetings)
So, the first thing that we would like this workflow to do when the cron node triggers, is to post a quick message letting everybody know that the coffee groups are about to be released. To do this, set the following parameters in the Mattermost node:
- Mattermost API credentials: Select your credentials that you set up earlier
- Resource: Message
- Operation: Post
- Channel ID: Your channel ID
- Message: 👋 Happy Monday! Groups for this week's virtual coffee are:
If you are not sure what the channel ID is for your Mattermost node, simply go to the title of the channel in the Mattermost page, click on the down chevron, and click on view info. This will pop up a new window telling you about your channel the channel ID is in fine print at the bottom left-hand corner of the window copy and paste this long string of letters into the channel ID field in the Mattermost node window.
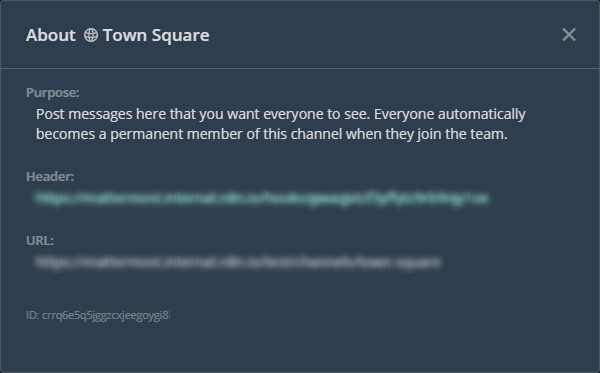
You are now ready to test your Mattermost node click on the execute node button at the top right-hand corner of the node window. You should now see the contents of your message field posted in the appropriate Mattermost channel.
You can rename the Mattermost node to Greetings.
Second Mattermost Node (Employees in Coffee Chat Channel)
The next Mattermost node will get a complete list of everybody who is in the channel that you chose in the previous node. The difference between this node and the previous Mattermost node that you created is that this node is being used to retrieve information whereas the previous node was used to post information. To configure this node, enter the following into the Mattermost node fields:
- Mattermost API credentials: Select your credentials that you set up earlier
- Resource: User
- Operation: Get All
- Return all: On
You will also want to add a field below the standard Mattermost fields. Click the add field dropdown button and select In Channel from the dropdown menu. In this field, use the Expression Editor to enter {{$node["Greetings"].parameter["channelId"]}}
so that the same channel ID is used as in the previous Mattermost node.
Please note that you may receive an error message indicating that you do not have appropriate permissions on the server. This will not affect the results of this workflow.

You can now test the second Mattermost node. Click the Execute Node button at the top right-hand corner. You should get a list of all the users that are present in the channel along with some extra information.
Once you have successfully retrieved this information you can rename the node Employees in Coffee Chat Channel and close the node.
Function Node
The function node is now going to be used to perform a few things:
- Put all users into an array
- Shuffle the list of channel users
- Break them up into groups of three
- If there is a group of just one, added to the previously created group
- Forward the groups on to the next nodes
Since reading function node can be some of the most complicated parts of writing a workflow, we are going to just provide you with the contents of this function node so that you can quickly get moving on to the next step. Here is the code:
const ideal_group_size = 3;
let groups = [];
let data_as_array = [];
let newItems = [];
// Take all the users and add them to an array
for (let j = 0; j < items.length; j++) {
data_as_array.push({username: items[j].json.username, email: items[j].json.email});
}
// Fisher-Yates (aka Knuth) Shuffle
function shuffle(array) {
let currentIndex = array.length, temporaryValue, randomIndex;
// While there remain elements to shuffle...
while (0 !== currentIndex) {
// Pick a remaining element...
randomIndex = Math.floor(Math.random() * currentIndex);
currentIndex -= 1;
// And swap it with the current element.
temporaryValue = array[currentIndex];
array[currentIndex] = array[randomIndex];
array[randomIndex] = temporaryValue;
}
return array;
}
// Randomize the sequence of names in the array
data_as_array = shuffle(data_as_array);
// Create groups of ideal group size (3)
for (let i = 0; i < data_as_array.length; i += ideal_group_size) {
groups.push(data_as_array.slice(i, i + ideal_group_size));
}
// Make sure that no group has just one person. If it does, take
// one from previous group and add it to that group
for (let k = 0; k < groups.length; k++) {
if (groups[k].length === 1) {
groups[k].push(groups[k-1].shift());
}
}
for (let l = 0; l < groups.length; l++) {
newItems.push({json: {groupsUsername: groups[l].map(a=> a.username), groupsEmail: groups[l].map(b=> b.email)}})
}
return newItems;
Copy and paste this code into the JavaScript code field in the function node. Rename the function node Divide into groups.
You can test to see if the code in the function node is working properly by pressing the Execute Node button at the top right-hand corner of the node.
You should get several array sets of three users each as your output. There is a chance that the last array will have two or four members in the array if the number of users in the channel is not evenly divisible by three. Close the function node.
Third Mattermost Node (Announce Groups)
The next thing that we are going to do is send out a post with the names of the people who will be getting together in the coffee groups.
Fill in the following parameters in this Mattermost node:
Mattermost API credentials: select the credentials that were provided earlier
- Mattermost API credentials: select the credentials that were provided earlier
- Resource: message
- Operation: post
- Channel ID: the channel that you used previously
- Message: groups to be distributed
For the information to go out properly, the message field will have to be filled out with an expression. Use the expression editor next to the message field and paste in the following:
☀️ {{$node["Divide into groups"].json["groupsUsername"].join(', ')}}
This will pull the user groups from the function node and use their information. Close the expression editor.
You can test your latest Mattermost node by clicking the Execute Node button at the top right corner. If this worked properly, you will be able to see the coffee groups appear in the appropriate Mattermost channel. You can rename the Mattermost node to Announce groups and close the node.
Google Calendar Node
The final thing we want to do with this workflow is to send out calendar invites to everyone in the groups. The Google calendar node will perform this for us.
Connect the Google Calendar node to the Divide into groups function node and set the following parameters:
- Google Calendar OAuth credentials: select your Google calendar credentials or set them up if this is the first time you have used them
- Resource: event
- Operation: create
- Calendar ID: your calendar ID
- Start: start date and time
- End: end date and time
- Use default reminder: on
We also want to use some additional fields in this node. Select and set the following additional fields using the add field dropdown button:
- Attendees: use the expression editor and add the following for the expression:
{{$node["Divide into groups"].json["groupsEmail"].join(',')}}
- Conference Data:
- Conference Link:
- Type: Google Meet
- Conference Link:
- Guests Can Modify: On
- Summary: n8n coffee catchup
You can test this node by clicking on the Execute Node button at the top right corner.
If everything works properly, each of the attendees should see a new calendar request in their calendar.
You can rename the node Send Calendar Invites and close the node.
At this point, please save and active your node so that it runs on schedule! Your final result should look like this:
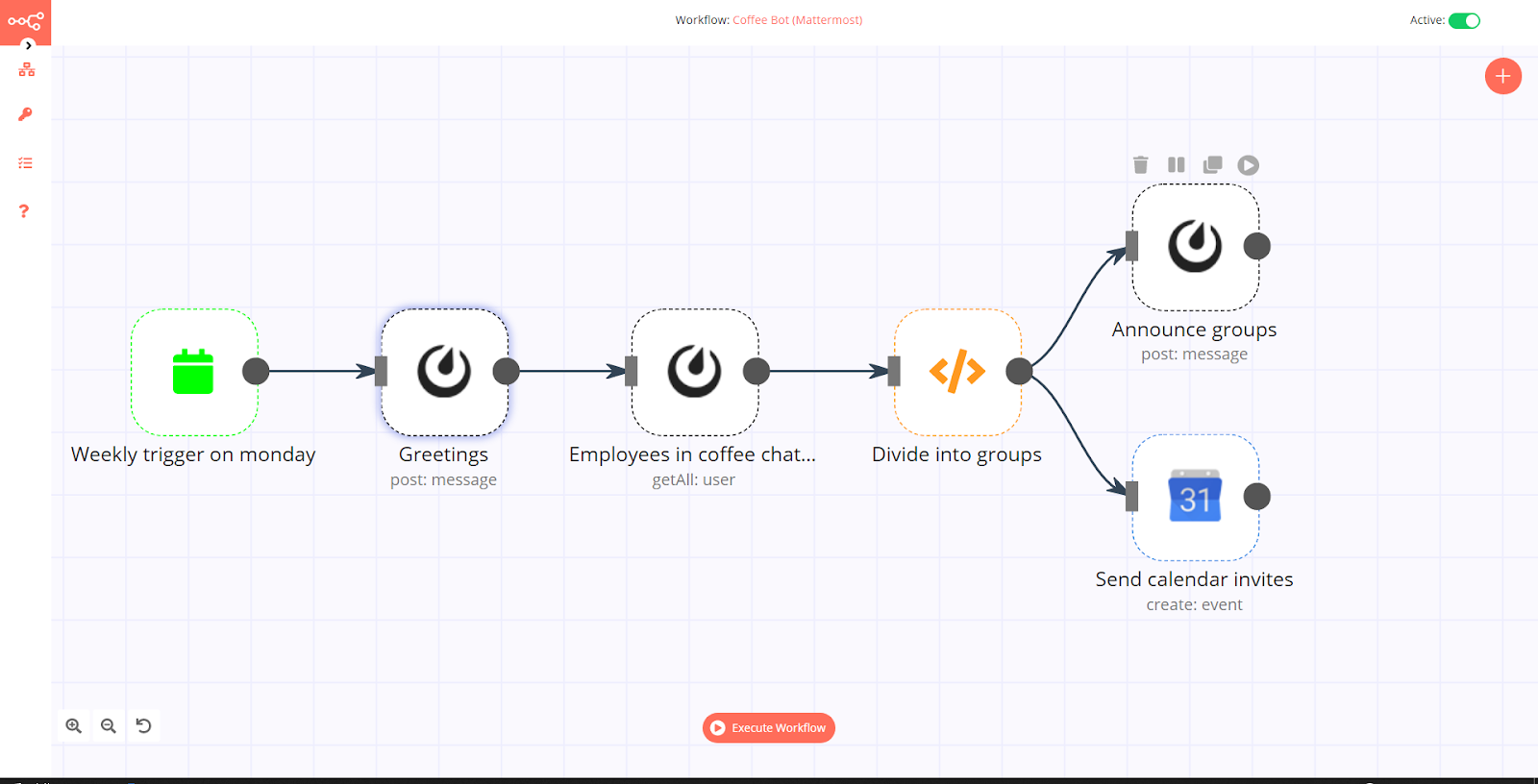
If everything goes according to plan, every Monday at 10:00 AM you will get an invitation for coffee.
Different Technology
Now if your new school or workplace uses Mattermost as their chat system, you're all set and ready to go. But, there are many different chat systems out there other than just Mattermost. Fortunately, n8n has you covered. It is simply a matter of replacing the Mattermost nodes with the comparable node from the other chat systems and fine-tuning the function node to support this new information.
Substituting Matrix
To use the Matrix messaging system rather than the Mattermost messaging system, follow these steps:
- Delete the 3 Mattermost nodes from the workflow and replace them with Matrix nodes with the same names and connect them the same way they were connected in the Mattermost workflow.
- Configure the Greetings node with the following settings:
- Matrix API Credentials: Your credentials that you had previously set up
- Resource: Message
- Operation: Create
- Room ID: This should be the room name that you are using for the coffee chat meetings
- Text: 👋 Happy Monday Groups for this week's virtual coffee are:
- Message Type: Text
- Message Format: Plain Text
- Configure the Employees in coffee chat channel node with the following settings:
- Matrix API Credentials: Your credentials that you had previously set up
- Resource: Room Member
- Operation: Get All
- Room ID: This should be the room name that you are using for the coffee chat meetings
- Filters:
- Membership: Any
- Update the Divide into groups function node with the following code so that it accepts the different output from the Matrix node and it does not look for email addresses (since Matrix does not supply them):
const ideal_group_size = 3;
let groups = [];
let data_as_array = [];
let newItems = [];
// Take all the users and add them to an array
for (let j = 0; j < items.length; j++) {
data_as_array.push({username: items[j].json.user_id});
}
// Fisher-Yates (aka Knuth) Shuffle
function shuffle(array) {
var currentIndex = array.length, temporaryValue, randomIndex;
// While there remain elements to shuffle...
while (0 !== currentIndex) {
// Pick a remaining element...
randomIndex = Math.floor(Math.random() * currentIndex);
currentIndex -= 1;
// And swap it with the current element.
temporaryValue = array[currentIndex];
array[currentIndex] = array[randomIndex];
array[randomIndex] = temporaryValue;
}
return array;
}
// Randomize the sequence of names in the array
data_as_array = shuffle(data_as_array);
// Create groups of ideal group size (3)
for (let i = 0; i < data_as_array.length; i += ideal_group_size) {
groups.push(data_as_array.slice(i, i + ideal_group_size));
}
// Make sure that no group has just one person. If it does, take
// one from previous group and add it to that group
for (let k = 0; k < groups.length; k++) {
if (groups[k].length === 1) {
groups[k].push(groups[k-1].shift());
}
}
for (let l = 0; l < groups.length; l++) {
newItems.push({json: {groupsUsername: groups[l].map(a=> a.username)}})
}
return newItems;
Configure the Announce groups node with the following settings:
- Matrix API Credentials: Your credentials that you had previously set up
- Resource: Message
- Operation: Create
- Room ID: This should be the room name that you are using for the coffee chat meetings
- Text: Use the Expression editor to enter the following expression:
☀️ {{$node["Divide into groups"].json["groupsUsername"].join(', ')}}
- Message Type: Text
- Message Format: Plain Text
- Delete the Calendar node. Since Matrix does not provide email addresses, there is nowhere to send out a calendar invite. (Maybe a shared Google Sheet with everyone’s account name and email address could be used as a lookup table in future versions! 🤔)
- Don’t forget to activate the workflow so that it runs every Monday
Your final Matrix workflow should look like this:
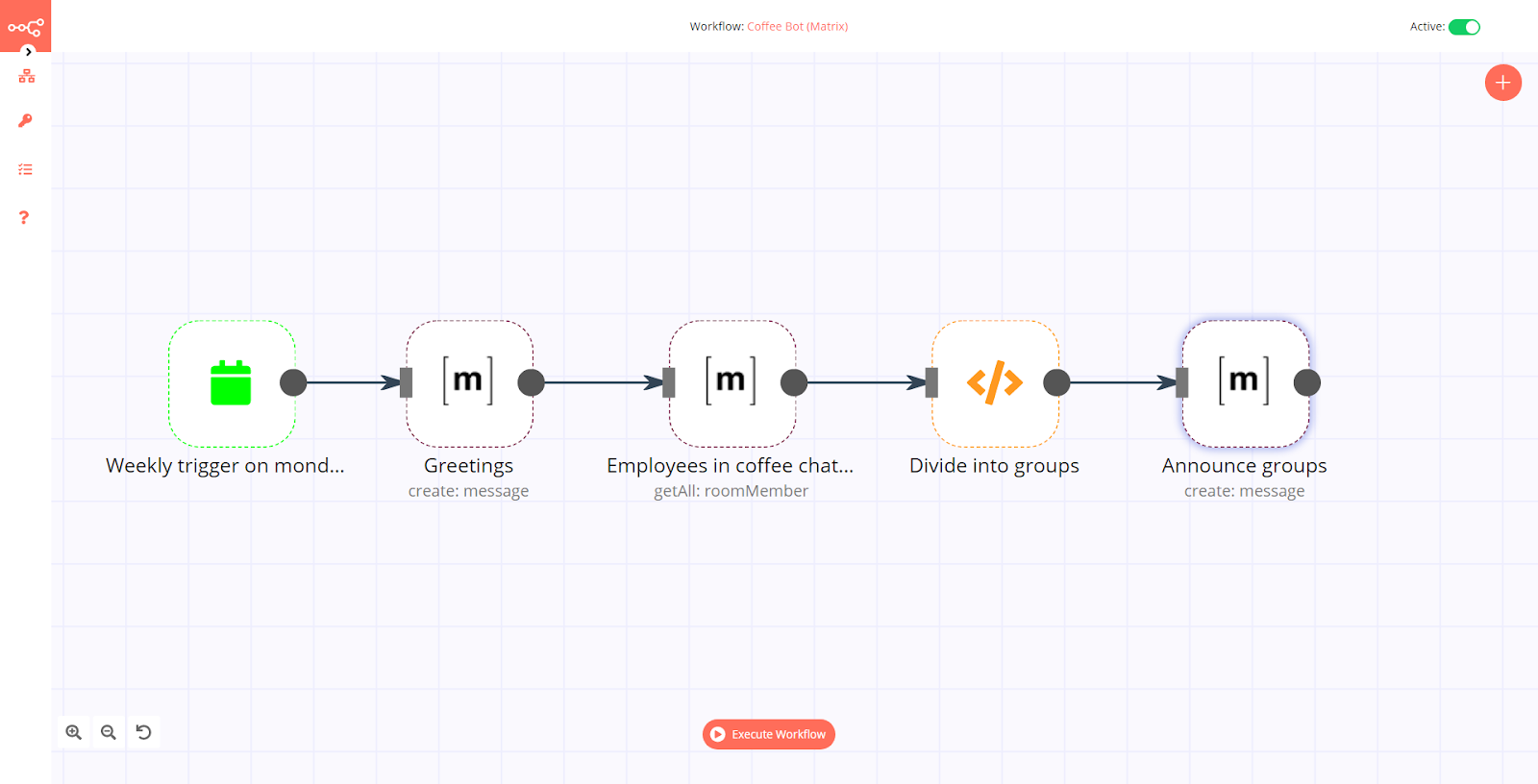
What’s Next?
Now that we have this basic coffee chat workflow running properly in different environments using different technologies, where could we take this? Here are some ideas:
- Send out calendar invites via the chat program using a calendar (ICS) file
- Integrate other chat technologies (e.g. Slack, Telegram, Teams, etc.)
- Create invitations to the actual video conference program (e.g. Zoom) and send the links
- Check people’s calendars and opt them out of the chat if they already have a meeting scheduled
Our Journey
We’ve covered a lot of ground today. Let’s review what we have accomplished:
- Built a workflow for creating random groups of three for a virtual meeting and sent out the groupings using Mattermost along with an invitation to their calendars
- Modified the workflow to use the Matrix chat program rather than Mattermost, taking into account differences in technology.
I’d love to hear about what you’ve built using n8n! Or if you’ve run into an issue while following the tutorial, feel free to reach out to me on Twitter or ask for help on our forum 💙
Start automating!
The best part is, you can start automating for free with n8n. The easiest way to get started is to sign up for a free n8n cloud trial. Thanks to n8n’s fair-code license, you can also self-host n8n for free.